Performance Check - innerHTML vs appendChild
3 min read • 21 Nov 2021Views:
We often use innerHTML and appendChild to add HTML Elements to the markup. But how these two differ? Which is better? Which is more performant?
Let's check this, creating a demo markup for the following analysis:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Performance Check: innerHTML vs appendChild</title>
</head>
<body>
<h1>Performance Check</h1>
<h3>innerHTML vs appendChild</h3>
<main></main>
<script src="main.js"></script>
</body>
</html>
For the analysis, we will set innerHTML and appendChild 1000 times, so that we get some comparable timestamps.
innerHTML
As per MDN, innerHTML gets or sets the HTML or XML markup contained within the element.
Performance of innerHTML
Code
const mainBox = document.querySelector("main");
const LIMIT = 1000;
console.time("innerHTML");
for (let i = 0; i < LIMIT; i++) {
mainBox.innerHTML += `<div>innerHTML - ${i}</div>`;
}
console.timeEnd("innerHTML");
5 Attempts with innerHTML
innerHTML Results
Sr. No. | Time taken |
---|---|
1 | 1665.57 ms |
2 | 1782.49 ms |
3 | 1546.03 ms |
4 | 1490.70 ms |
5 | 1899.51 ms |
-------- | ---------- |
Average | 1676.86 ms |
appendChild
As per MDN, The appendChild method adds a node to the end of the list of children of a specified parent node.
Performance of appendChild
Code
const mainBox = document.querySelector("main");
const LIMIT = 1000;
console.time("appendChild");
for (let i = 0; i < LIMIT; i++) {
const el = document.createElement("div");
el.innerText = `appendChild - ${i}`;
mainBox.appendChild(el);
}
console.timeEnd("appendChild");
5 Attempts with appendChild
appendChild Results
Sr. No. | Time taken |
---|---|
1 | 5.54 ms |
2 | 3.81 ms |
3 | 3.98 ms |
4 | 4.35 ms |
5 | 4.84 ms |
-------- | ---------- |
Average | 4.50 ms |
Conclusion
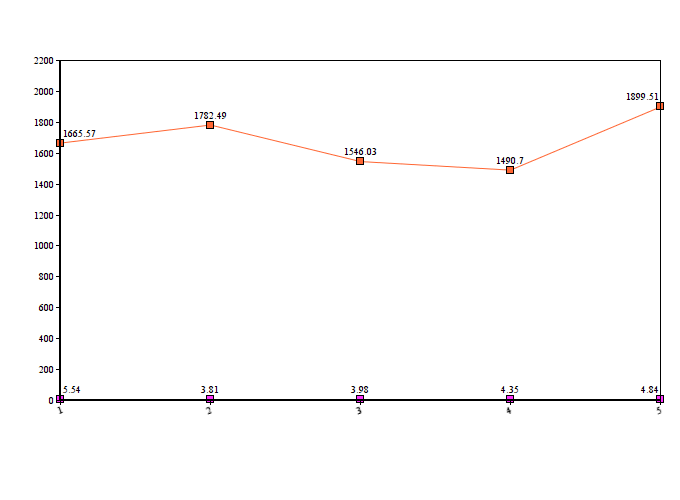
what's the difference?
- If an element (say el) markup is set using innerHTML, it will rebuild all the DOM element's inside that element (el).
- If an element (say el) markup is set by adding child using appendChild, it will only build that particular element node and append it. Saving the performance in rebuilding all the DOM element's inside that element (el).
when to use?
- innerHTML: innerHTML can become the perfect choice when the need is to set markup once, and that markup is large. We can also insert data using string interpolation.
- appendChild: appendChild can be very performant when the need is to append children in a loop.